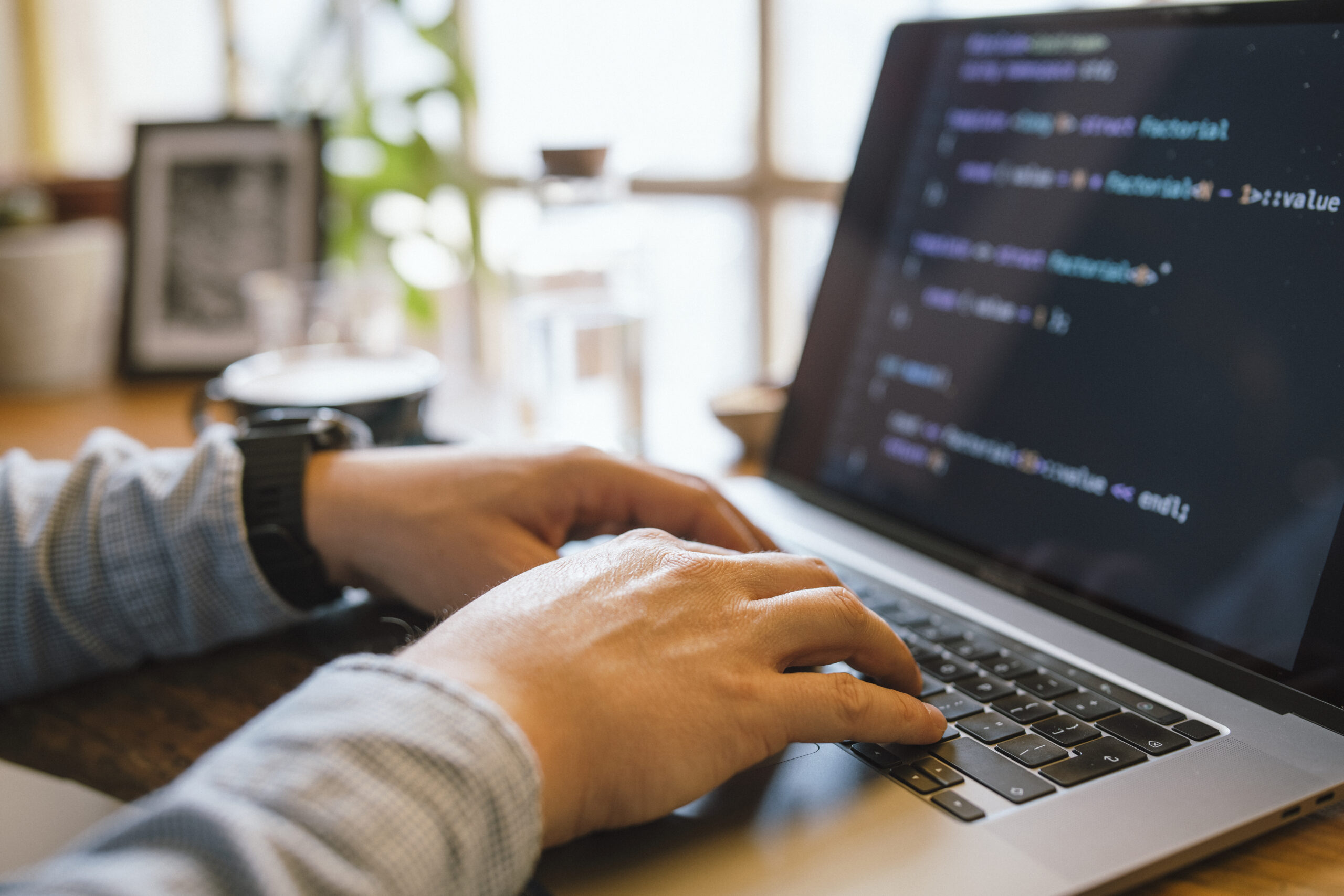
Debugging is Among the most important — still normally neglected — competencies in a developer’s toolkit. It is not nearly repairing broken code; it’s about knowledge how and why matters go wrong, and Finding out to Consider methodically to resolve troubles successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person Component of advancement, understanding how to connect with it properly throughout execution is Similarly critical. Modern day development environments appear Outfitted with potent debugging abilities — but numerous builders only scratch the surface area of what these tools can perform.
Consider, such as, an Integrated Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and even modify code to the fly. When utilized effectively, they Allow you to notice precisely how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They assist you to inspect the DOM, check community requests, view true-time performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can flip discouraging UI issues into manageable jobs.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about running processes and memory management. Learning these resources could possibly have a steeper learning curve but pays off when debugging functionality difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Command systems like Git to know code historical past, uncover the precise minute bugs were being introduced, and isolate problematic adjustments.
Eventually, mastering your instruments suggests likely further than default configurations and shortcuts — it’s about acquiring an personal expertise in your development environment in order that when concerns come up, you’re not dropped at nighttime. The higher you understand your resources, the more time you are able to invest solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes disregarded — actions in efficient debugging is reproducing the problem. Right before leaping to the code or producing guesses, developers have to have to produce a regular surroundings or scenario where the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of possibility, frequently bringing about wasted time and fragile code modifications.
Step one in reproducing an issue is accumulating as much context as possible. Check with inquiries like: What actions brought about the issue? Which natural environment was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the easier it will become to isolate the exact ailments underneath which the bug occurs.
As you’ve collected more than enough details, seek to recreate the challenge in your neighborhood surroundings. This may suggest inputting a similar info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, look at writing automated assessments that replicate the edge circumstances or point out transitions involved. These exams not simply aid expose the situation but also avoid regressions Down the road.
Sometimes, The problem can be environment-certain — it would materialize only on particular working programs, browsers, or less than particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a move — it’s a state of mind. It needs persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you are presently halfway to repairing it. That has a reproducible state of affairs, you can use your debugging tools much more successfully, check prospective fixes securely, and talk much more Obviously together with your group or customers. It turns an abstract criticism right into a concrete challenge — and that’s where builders prosper.
Read through and Fully grasp the Mistake Messages
Error messages are frequently the most respected clues a developer has when some thing goes Incorrect. Rather than looking at them as discouraging interruptions, builders must find out to treat mistake messages as immediate communications through the program. They generally inform you what exactly took place, where by it happened, and sometimes even why it happened — if you know how to interpret them.
Begin by reading the information thoroughly As well as in entire. Several developers, especially when under time pressure, glance at the 1st line and quickly commence generating assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines like yahoo — read and fully grasp them initial.
Crack the error down into sections. Could it be a syntax error, a runtime exception, or perhaps a logic mistake? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically adhere to predictable designs, and Discovering to recognize these can drastically accelerate your debugging course of action.
Some errors are obscure or generic, As well as in Those people instances, it’s critical to look at the context in which the error transpired. Test related log entries, input values, and recent improvements during the codebase.
Don’t neglect compiler or linter warnings both. These typically precede larger sized issues and provide hints about prospective bugs.
In the long run, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, serving to you pinpoint challenges quicker, minimize debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an application behaves, helping you comprehend what’s happening under the hood without needing to pause execution or step through the code line by line.
A very good logging system starts off with recognizing what to log and at what stage. Prevalent logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic data for the duration of advancement, Information for common occasions (like successful start-ups), Alert for likely concerns that don’t break the applying, ERROR for actual complications, and Lethal once the method can’t go on.
Prevent flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your procedure. Center on crucial activities, state improvements, input/output values, and important determination points as part of your code.
Format your log messages Evidently and persistently. Include context, for instance timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
In the end, clever logging is about balance and clarity. By using a perfectly-believed-out logging technique, you can reduce the time it will require to identify problems, achieve further visibility into your purposes, and improve the Total maintainability and trustworthiness of your code.
Consider Similar to a Detective
Debugging is not only a technological job—it is a method of investigation. To successfully recognize and take care of bugs, developers need to tactic the procedure like a detective solving a thriller. This way of thinking helps break down intricate challenges into manageable elements and comply with clues logically to uncover the root trigger.
Start off by accumulating proof. Think about the symptoms of the trouble: error messages, incorrect output, or functionality difficulties. Just like a detective surveys a crime scene, gather as much relevant info as you are able to with no jumping to conclusions. Use logs, examination circumstances, and user reviews to piece with each other a transparent photo of what’s occurring.
Upcoming, sort hypotheses. Question on your own: What may very well be resulting in this behavior? Have any modifications lately been made into the codebase? Has this challenge transpired just before below similar instances? The target is usually to slim down possibilities and detect probable culprits.
Then, examination your theories systematically. Attempt to recreate the problem in a very controlled environment. For those who suspect a certain perform or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, request your code questions and Permit the outcomes guide you closer to the reality.
Shell out close awareness to tiny aspects. Bugs typically hide from the least predicted locations—similar to a missing semicolon, an off-by-just one error, or maybe a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly knowing it. Non permanent fixes could disguise the real dilemma, just for it to resurface later.
And lastly, keep notes on Whatever you tried and realized. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and enable Other individuals fully grasp your reasoning.
By thinking just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden concerns in advanced systems.
Create Exams
Producing checks is among the most effective methods to increase your debugging techniques and In general development efficiency. Exams not just support capture bugs early and also function a security net that gives you self-confidence when producing adjustments to the codebase. A properly-examined software is simpler to debug as it lets you pinpoint particularly wherever and when a dilemma takes place.
Begin with unit assessments, which center on unique capabilities or modules. These smaller, isolated assessments can promptly expose no matter whether a certain piece of logic is Operating as expected. When a exam fails, you straight away know wherever to glance, drastically minimizing enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—troubles that reappear right after previously being fastened.
Following, integrate integration checks and conclusion-to-conclude exams into your workflow. These help make sure several areas of your application get the job done collectively smoothly. They’re significantly valuable for more info catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to think critically about your code. To check a attribute properly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug can be a powerful initial step. As soon as the check fails continually, you are able to target correcting the bug and view your examination go when the issue is settled. This technique makes certain that exactly the same bug doesn’t return Later on.
Briefly, writing tests turns debugging from a discouraging guessing activity into a structured and predictable method—supporting you capture extra bugs, quicker plus much more reliably.
Choose Breaks
When debugging a tough problem, it’s straightforward to become immersed in the challenge—gazing your monitor for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your thoughts, minimize stress, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for too lengthy, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less efficient at trouble-resolving. A short walk, a coffee crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, Specially in the course of longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Strength along with a clearer mentality. You would possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, having breaks just isn't an indication of weakness—it’s a smart tactic. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing useful in case you make the effort to replicate and review what went wrong.
Begin by asking oneself a number of critical issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit tests, code evaluations, or logging? The solutions usually reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding routines moving ahead.
Documenting bugs will also be a wonderful practice. Retain a developer journal or retain a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. Eventually, you’ll begin to see patterns—recurring concerns or typical mistakes—you could proactively stay clear of.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be Primarily highly effective. Whether or not it’s via a Slack concept, a short generate-up, or a quick understanding-sharing session, encouraging Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a few of the most effective developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, each bug you correct provides a fresh layer on your skill set. So upcoming time you squash a bug, take a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and patience — nevertheless the payoff is large. It makes you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at Anything you do.